-
About Python Programming Language
-
History of Python
-
Characteristics of Python
Tutors
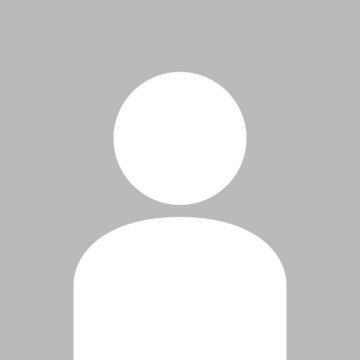
eKalasaala
PROGRAM OVERVIEW
It was created by Guido van Rossum during 1985- 1990.
By using Python Language we can implement different variety of applications like
1. Automation Applications.
2. Data Analysis.
3. Web Applications.
4. Scientific Obligations.
5. Web Scraping Techniques.
6. Network with IOT Application.
7. Administration Scripts.
8. Test Cases.
9. GUI Applications.
10. Gaming Applications.
11. Animation Applications.......etc.
Currently copy rights of the python language are registered with an open community and non-profitable organization called Python Software foundation (PSF). Python language is open source and source code is also open source. The Python Software which is developed by the Python Software Foundation is known as 'CPython'. There are so many distributions available for CPython. CPython is open source and platform independent. With respect to every operating system separate python software'
CURRICULUM
Introduction to Python Programming
Python Environment
-
Installation of Python
-
Compile and Running
Editors & IDE's
-
Introduction to IDE
-
PyCharm
-
Running Python Script
Getting Started with Python Programming
-
Creating your First Python Program
-
Reading & Printing to Screen
-
Interactive & Script Mode
-
Python File Extensions
Basic Concepts in Python
-
Shell as a Calculator
-
Comments in Python
-
Multiline Statements
-
Quotations in Python
Variables
-
Introduction to Variables
-
Naming Variables
-
Mnemonic Variable Names
-
Reserved Words
Data Types
-
Values & Types
-
Numerical Data Type
-
String Data Type
Working with Variables
-
Multiple Assignment
-
Swap Variables
-
Type Conversion
-
Mutable vs Immutable Objects
-
Number System Conversion
Operators
-
Arithmetic Operator
-
Assignment Operator
-
Comparison Operator
-
Logical Operator
-
Bitwise Operator
-
Membership Operator
-
Operator Precedence
-
Evaluating Expression
Decision Making
-
Introduction to Decision Making
-
if Statement
-
if...else Statement
-
elif Statement
-
Nested if...else Statement
Loops
-
Introduction to Loops
-
While Loop
-
For Loop
-
Nested Loops
-
Break Statement
-
Continue Statement
-
Pass Statement
List
-
Introduction to List
-
Accessing Items in a List
-
Loop through List
-
List Length & Add Items to List
-
Built-in List Methods
-
Basic List Operations
-
List Mutability
Tuples
-
Introduction to Tuple
-
Tuple Packing & Unpacking
-
Comparing Tuples & Iterating through Tuple
-
Deleting & Slicing of Tuples
-
Tuple Membership Test
Functions
-
Introduction to Functions
-
Defining & Calling a Function
-
Working of Function
-
Doc Strings
Functions : Arguments & Return Statements
-
Default Arguments
-
Required Arguments
-
Keyword Arguments
-
Variable Length Arguments
-
Return Statement
-
Returning Multiple Values
-
Command Line Arguments
Functions : Scope of Variables
-
Global Variable
-
Local Variable & Its Comparison with Global
Functions : Recursion
-
Recursive Functions
-
Finding Sum of Natural Numbers using Recursion
NumPy Package
-
Introduction to NumPy
-
Installation of NumPy
-
Working with NumPy
-
Data Types in NumPy
-
NumPy Array Attributes
Basics of Matrices
-
Creating a Matrix
-
Identity Matrix
-
Transpose of a Matrix
Matrix Arithmetic Operations
-
Matrix Addition
-
Matrix Subtraction
-
Matrix Multiplication
-
Matrix Element Multiplication & Division
Operations on Matrices
-
Determinant of a Matrix
-
Inverse of a Matrix
-
Solving Linear Equations
Exercises on Matrices
-
Picking an Element from a Matrix
-
Interchange Diagonal Elements
-
Check a Matrix is Identity or Not
-
Matrix Arithmetic Operations
-
Inverse of 2X2 & 3X3 Matrix
-
Solving Linear Equations
Transpose of a Matrix without using NumPy
-
Introduction to Transpose of Matrix
-
Function to Input a Matrix : Code Explanation
-
Function to Input a Matrix : Code Implementation
-
Function to Transpose a Matrix : Code Explanation
-
Function to Transpose a Matrix : Code Implementation
-
Comparing with In-Built Function in NumPy
Determinant of Matrix without using NumPy
-
Manually Calculating Determinant of Matrix
-
Laplace Expansion to Calculate Determinant
-
Function to Find Determinant : Code Explanation
-
Function to Find Determinant : Code Implementation
-
Comparing with In-Built Function in NumPy
Cofactor & Minor Matrix without using NumPy
-
Introduction to Cofactors & Minor Matrix
-
Function for Cofactors & Minor Matrix : Code Explanation
-
Function for Cofactors & Minor Matrix : Code Implementation