-
Programming Languages Paradigm
-
Imperative Paradigm
-
Declarative Paradigm
-
Structured Programming
-
Procedural Programming
-
Object Oriented Programming
-
Functional Programming
Tutors
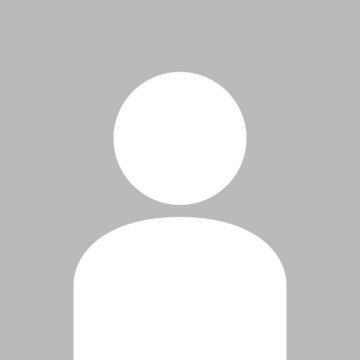
eKalasaala
PROGRAM OVERVIEW
CURRICULUM
Introduction to Programming Languages
History of Programming Language
-
History of Programming Language
Classification of Programming Languages
-
Low Level Programming Language
-
Middle Level Programming Language
-
High Level Programming Language
High level programming languages
-
FORTRAN
-
ALGOL
-
COBOL
-
BCPL
-
PASCAL
-
B Language
-
C Language
-
C++
-
Java
-
C#
Introduction to C#
-
Why it is Named as C#
-
Is C# Platform Dependent or Independent
Features of C#
-
Introduction to Features of C#
-
Simple
-
Modern Programming Language
-
Object Oriented
-
Type Safe
-
Interoperability
-
Scalable and Updatable
-
Component Oriented
-
Structured Programming Language
-
Rich Library
-
Fast Speed
Versions of C#
-
Versions of C#
Importance of C#
-
Advantages of C#
-
What will you gain if you learn C#?
History of C#
-
Beginning of Modern Age Programming
-
Creation of OOPS and C++
-
Internet and Java Emerge
-
Creation of C#
-
Evolution of C#
-
How C# Relates to the .NET Framework
-
.NET Framework and its Components
Your First Program
-
First Program using Command Line Compiler
-
First Program Using Visual Studio IDE
-
Handling Syntax Errors
-
Debugging
Basic Syntax
-
Semicolons
-
Keywords
-
Identifiers
Data Types
-
Introduction to Data Types
-
Different Data Types in C#
-
Value Data Type
-
Reference Data Type
-
Pointer Data Type
-
Why Data Types are Important
Literals & Variables
-
Literals
-
Variables
-
Initializing Variables
-
Scope of Variables
Operators
-
Introduction to Operators
-
Arithmetic Operator
-
Increment & Decrement Operator
-
Bitwise Operator
-
Ternary Operator
-
Spacing & Parenthesis
-
Operator Precedence
Conditional Statements
-
If Statement
-
If-else Statement
-
If-else if Statement
-
Nested if-else Statement
-
Switch Statement
-
Nested Switch Statement
Jump Statements
-
goto Statement
-
break Statement
-
continue Statement
-
return Statement
Iteration Statements
-
While Statement
-
Do-while Statement
-
For Statement
-
For each Statement
-
Some Variations on For Loop
Overview of OOPS
-
Introduction to OOPS
-
Class
-
Object
-
Abstraction
-
Encapsulation
-
Inheritance
-
Polymorphism
Classes
-
Introduction to Class
-
Rules for Declaring Class Name
-
Access Specifiers or Modifiers
-
Generalized program for Access Specifier or Modifier
-
Internal & Protected
-
Generalized program for a Class
Objects
-
Introduction to Object
-
Creating an Object
-
Releasing an Object
-
Declaring & Instantiating Object
-
Access to fields of an Object
Methods
-
Introduction to method
-
Method Deceleration
-
Return Type Method
-
Non - Return Type Method
Types of Methods
-
Pure Virtual Method
-
Virtual Method
-
Abstract Method
-
Partial Method
-
Extension Method
-
Instance Method
-
Static Method
Passing Parameter to a Method
-
Introduction to Passing Parameter to a Method
-
Value as a Parameter
-
Reference as a Parameter
-
Output as a Parameter
Method Overloading
-
Introduction to Method Overloading
-
By Changing the Number of Parameters
-
By Changing the Data Types of the Parameters
-
By Changing the Order of the Parameters
Constructors
-
Introduction to Constructor
-
Rules for Declaring a Constructor
Types of Constructor
-
Default Constructor
-
Parametarized Constructor
-
Copy Constructor
-
Private Constructor
-
Static Constructor
Destructors
-
Introduction to Destructor
-
Rules for Declaring a Destructor
-
Garbage Collector