-
Procedure Oriented Paradigm
-
OOPS Paradigm
-
Basic Concepts of OOPS
-
Benefits of OOPS
-
Features of OOPS
Tutors
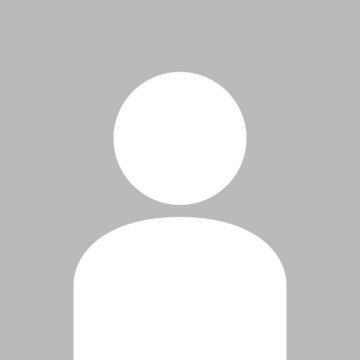
eKalasaala
PROGRAM OVERVIEW
It is therefore possible to code C++ in a "C style" or "object-oriented style." In certain scenarios, it can be coded in either way and is thus an effective example of a hybrid language.
C++ is considered to be an intermediate-level language, as it encapsulates both high- and low-level language features. Initially, the language was called "C with classes" as it had all the properties of the C language with an additional concept of "classes." However, it was renamed C++ in 1983.
Data structure, way in which data are stored for efficient search and retrieval. Different data structures are suited for different problems. Some data structures are useful for simple general problems, such as retrieving data that has been stored with a specific identifier. For example, an online dictionary can be structured so that it can retrieve the definition of a word.
CURRICULUM
Overview of Object Oriented Programming System (OOPS) Basics
Introduction to C++
-
Overview of C++
-
Programming Structure
Installation Procedure
-
Installation Procedure of Code Block IDE
-
Hello World Program
Tokens
-
Data Types
-
Keywords
-
Variables and Identifiers
-
Literals
-
Constants
-
Operators
Input and Output Streams
-
cout
-
cin
-
cerr
-
clog
Variable Declaration
-
Variables and Datatypes
-
Modifiers
-
Qualifiers
C++ Programs on Variable Declaration
-
Program on Constant Variable
Storage Class Specifiers
-
Introduction to Storage Class Specifiers
-
Local Variables
-
Static Variables
Operators
-
Introduction to Operators
-
Unary Operators
-
Binary Operators
-
Bitwise Operators
-
Ternary and Special Operators
C++ Programs on Operators
-
Program on Unary Operators
-
Program on Arithmetic Operators
-
Program on Relational Operators
-
Program on Logical Operators
Conditional Statements
-
Branching Statements
-
if Statement
-
if..else Statement
-
Nested Statements
-
switch Statement
C++ Programs on Conditional Statements
-
Program on if Statement
-
Program on if..else Statement
-
Program on Nested Statements
-
Program on switch Statement
Loops : while Loop
-
Introduction to while Loop
-
Program to Print 1 to 10 Numbers using while Loop
C++ Programs on while Loop
-
Program to Print Sum of Numbers from 1 to 10 using while Loop
-
Program to Print Multiplication Table of 2 using while Loop
Loops : do.. while Loop
-
Introduction to do..while Loop
-
Program to Print 1 to 10 Numbers using do..while Loop
Loops : for Loop
-
Introduction to for Loop
-
Program to Print 1 to 10 Numbers using for Loop
C++ Programs on for Loop
-
Program to Print Sum of Numbers From 1 to 10 using for Loop
Loops : Break and Continue Statements
-
Introduction to Break Statement
-
Introduction to Continue Statement
C++ Programs on Break and Continue Statements
-
Program on Break Statement
-
Program on Continue Statement
Loops : Nested Loops
-
Nested for Loop
C++ Programs on Nested Loops
-
Program on Nested for Loop
Arrays
-
Introduction to Arrays
-
Accessing Elements of an Array
-
One Dimensional Array
-
Two Dimensional Array
-
Difference Between 1-Dimensional and 2-Dimensional Array
C++ Programs on Arrays
-
Program to Find Sum of 5 Input Numbers
-
Program to Add Same Size of Two Matrices
-
Program to Read Values into 2 Dimensional Array
Pointers
-
Introduction to Pointers
-
Pointer Variables
-
Usage of Pointers
-
Double Pointer
-
Pointer to an Array
C++ Programs on Pointers
-
Program to Find the Address of a Given Integer without using Pointers
-
Program to find the Address of a Given Integer using Pointers
-
Program on Double Pointer
-
Program on Pointer which is Pointing Address of an Integer
-
Program to Subtract Two Pointers Containing Same Array
-
Program on Pointer to an Array of 5 Integers
Strings
-
Introduction to Strings
-
String Class Type
-
String Class Type versus Character Array
C++ Programs on Strings
-
Program on How String can be Printed using Different Notations
Structures
-
Introduction to Structures
-
Array of Structures
C++ Programs on Structures
-
Program to Create Student Data Using Structure - Code Logic Explanation
-
Program to Create Student Data Using Structure - Code Logic Implementation
-
Program to Display all Students Information using Array of Structure
-
Program to Get a Student Data from a Pointer to Structure
Enumeration
-
Introduction to Enumeration(enum)
-
Program on Enumeration(enum)
Typedef
-
Introduction to Typedef
-
Program on Typedef to Understand the Types Assigned to Existing Types
-
Reference
Functions
-
Introduction to Functions
-
Program on Function - Logic Explanation
-
Defining a Function
C++ Programs on Functions
-
Introduction to Function Prototype
-
Program on Function without Parameter and with Return Value
-
Program on Function without Parameter and without Return Value
-
Program on Function with Parameter and without Return Value
-
Program on Function with Parameter and with Return Value
-
Actual and Formal Arguments
Functions : Parameter Passing Techniques
-
Introduction to Parameter Passing Techniques
-
Call by Value
-
Call by Address or Pointer
-
Call by Reference
-
Difference Between Call by Value, Address and Reference
-
Default Arguments
Functions : Function Overloading
-
Introduction to Function Overloading
-
Program on Sum of 2 and 3 Numbers
Functions : Recursive and Inline Functions
-
Introduction to Recursive Functions
-
Introduction to Inline Functions
-
Advantages and Disadvantages of Inline Function
C++ Programs on Recursive and Inline Functions
-
Program to Find Factorial of a Given Number using Recursion
-
Program on Fibonacci Sequence using Recursion
-
Program to Find Square Root of a Given Number
-
Program to Create Automatic Variables using Recursive Function
-
Program to Create Static Variables using Recursive Function
OOPS : Overview of OOPS
-
Introduction to OOPS
-
Introduction to Class
-
Syntax Explanation of Class
-
Introduction to Object
-
Program to Create Student Details using Class and Object
OOPS : Encapsulation and Abstraction
-
Introduction to Encapsulation
-
Introduction to Abstraction
C++ Programs on OOPS : Encapsulation and Abstraction
-
Program to Find Sum of Two Numbers using Encapsulation
-
Program to Get Student Data Abstraction by using Private Data
OOPS : Inheritance
-
Overview of Inheritance
-
Usage of Inheritance
C++ Programs on OOPS : Inheritance
-
Program on Inheritance from Animal to Dog and Cat - Code Logic Explanation
-
Program on Inheritance from Animal to Dog and Cat - Code Implementation
OOPS : Polymorphism
-
Introduction to Polymorphism
-
Introduction to Method Overloading
-
Introduction to Method Overriding
C++ Programs on OOPS : Polymorphism
-
Program on sum of Two Numbers using Method Overloading
-
Program on Method Overriding
OOPS : Static Keyword
-
Introduction to Static Keyword
-
Static Variable in Functions
-
Static Class Objects
-
Static Data Member in Class
-
Static Member Functions
C++ Programs on OOPS : Static Keyword
-
Program on Static Variable in Functions
OOPS : Binding
-
Introduction to Binding
-
Static Binding
-
Dynamic Binding
-
Message Passing
C++ Programs on OOPS : Binding
-
Program to Find Base Class Information using Static Binding
-
Program on Dynamic Binding using Virtual Functions
Classes and Objects
-
Class Declaration
-
Program to Print Name using Class and Object with Member Function
-
Program to Read and Display Student Information
Classes and Objects : Accessing Data Members
-
Accessing Data Members of Class
-
Accessing Private Data Members
-
Accessing Protected Data Members
C++ Programs on Classes and Objects : Accessing Data Members
-
Program on Accessing Data members using Objects
-
Program on Accessing Private Data Members using Public Member Function of Own Class
-
Program on Public, Private and Protected Data Members of a Class
Classes and Objects : Class Member Functions
-
Class Member Functions
-
Defining Function Outside the Class
-
Defining Function Inside the Class
-
Defining Members, Data Members and Methods
-
Difference Between Class and Structure
C++ Programs on Classes and Objects : Class Member Functions
-
Program on Use of Data Members, Members, and Methods using Class Members and Objects
Classes and Objects : Objects as Arguments
-
Introduction to Objects as Arguments
-
Passing Objects as an Argument to a Member Function - Code Logic Explanation
-
Passing Objects as an Argument to a Member Function - Code Logic Implementation
-
Returning Objects
-
Friend Function
C++ Programs on Classes and Objects : Objects as Arguments
-
Program on Returning Objects from Function
-
Program on Friend Function using with Class
Constructors
-
Introduction to Constructor
-
Default Constructor
-
Zero Parameterized Constructor
-
Parameterized Constructor
-
Copy Constructor
C++ Programs on Constructors
-
Program to Create Object using Constructor
-
Program on Default Constructor
-
Program to Find Student Information using Copy Constructor
Constructors : Dynamic Constructor and Constructor Overloading
-
Dynamic Constructor
-
Constructor Overloading
C++ Programs on Dynamic Constructor and Constructor Overloading
-
Program to Create Dynamic Memory During Runtime using New Keyword in Dynamic Constructor
-
Program on Constructor Overloading
Destructors
-
Introduction to Destructor
-
Constructor versus Destructor
C++ Programs on Destructors
-
Program on Destructor in Class
-
Program on Constructor and Destructor
-
Program to Create and Delete Dynamic Memory using New and Delete Keywords
Overloading : Function Overloading
-
Introduction to Overloading
-
Introduction to Function Overloading
C++ Programs on Function Overloading
-
Program to Print Values of Overloading Functions without using Class
-
Program to Print Values of Overloading Function using Class
Overloading : Unary Operator Overloading
-
Introduction to Operator Overloading
-
Operator Overloading Rules
-
Overloading Unary Operation
C++ Programs on Unary Operator Overloading
-
Program on Unary Operator Overloading using (!) Not Operator
-
Program on Unary Operator Overloading using (+) Operator
C++ Programs on Binary Operator Overloading
-
Program on Binary Operator Overloading using (+) Operator
-
Program on Binary Operator Over to Concatenate Two Strings - Code Logic Explanation
-
Program on Binary Operator Over to Concatenate Two Strings - Code Logic Implementation
-
Program to Implement Binary Operator Over Loading using Friend Function
-
Important Points for Overloading
Inheritance
-
Introduction to Inheritance
-
Purpose of Inheritance
-
Simple Program on Inheritance
Inheritance : Inheritance Visibility Mode
-
Introduction to Inheritance Visibility Mode
-
Public Inheritance
-
Private Inheritance
-
Protected Inheritance
C++ Programs on Inheritance Visibility Mode
-
Program on Private Inheritance
Inheritance : Types of Inheritance
-
Introduction to Single Inheritance
-
Ambiguity in Single Inheritance
-
Multi Level Inheritance
-
Multiple Inheritance
-
Hierarchical Inheritance
-
Hybrid Inheritance
C++ Programs on Inheritance Types
-
Program on Single Inheritance with Public Mode
-
Program on Single Inheritance with Private Mode
-
Program on Single Inheritance with Protected Mode
-
Program on Same Functions Present in Both Base and Derived Classes