-
Introduction to Programming Paradigms
-
Imperative Paradigm
-
Imperative Paradigm
Tutors
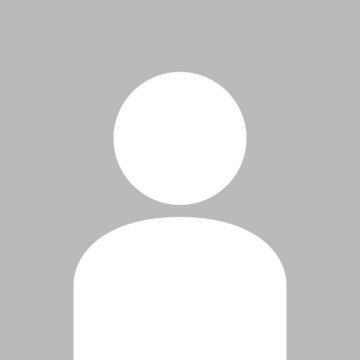
eKalasaala
PROGRAM OVERVIEW
C has proved to be a powerful and flexible language that can be used for a variety of applications, from business programs to engineering. C is a particularly popular language for personal computer programmers because it is relatively small -- it requires less memory than other languages.
The first major program written in C was the UNIX operating system, and for many years C was considered to be inextricably linked with UNIX. Now, however, C is an important language independent of UNIX.
Although it is a high-level language, C is much closer to assembly language than are most other high-level languages. This closeness to the underlying machine language allows C programmers to write very efficient code. The low-level nature of C, however, can make the language difficult to use for some types of applications.
Latest Version of C
The current lat
CURRICULUM
Programming Paradigms
Classifications of Programming Languages
-
Introduction to Classifications of Programming Languages
-
Low Level Languages
-
Middle Level Languages
-
High Level Languages
Programming Languages Before C
-
Introduction to Programming Languages before C
-
FORTRAN
-
ALGOL
-
COBOL
-
BCPL
-
PASCAL
-
B Language
-
C Language
About C Language
-
Evolution of C Language
-
What Language C was Written?
-
Characteristics of C
-
Is C Procedural Oriented Language?
Reasons to use C Language
-
Introduction to Reasons to use C Language
-
C Language Learning Outcomes
-
C as General Purpose Language
-
Standard Libraries in C
Introduction to C Language
-
Overview of C Language
-
Features of C
-
C Language Processing System
-
Memory Regions
-
Structure of C Program
Installation Procedure
-
Installation Procedure
Compilation and Execution
-
Introduction to Compilation and Execution
-
Errors During Compilation and Runtime
-
Compilation Errors
Tokens
-
Introduction to Tokens
-
Keywords
-
Constants
-
Identifiers
-
Special Symbols
-
Variables
-
Opearators
Data Types : Primary Data Types
-
Introduction to Data Types
-
Integer Data Types
-
Floating Point Data Types
-
Character Data Types
-
Void Data Types
Data Types : Secondary Data Types
-
Array and Pointer
-
Structure
-
Union
-
enum
Modifiers and Format Specifiers
-
Modifiers
-
Format Specifiers
First C Program using printf and scanf Functions
-
First C Program using printf Function
-
First C Program using scanf Function
Escape Sequence and Comments
-
Escape Sequence
-
Comments
Operators : Unary Operators
-
Introduction to Operators
-
Increment Operator
-
Decrement Operator
C Programs on Unary Operators
-
Program on Increment and Decrement Operators
Operators : Binary Operators
-
Introduction to Arithmetic Operators
-
Introduction to Assignment Operators
-
Relational Operators
-
Logical Operators
-
Introduction to Bitwise Operators
C Programs on Binary Operators
-
Program on Arithmetic Operators
-
Program on Assignment Operators
-
Program on Bitwise Operators
Operators : Ternary and Special Operator
-
Ternary Operator
-
Comma and sizeof Operator
C Programs on Ternary and Special Operators
-
Program on Special Operators
Operators : Operator Precedence and Evaluation
-
Precedence Relation
-
List of Operator Precedence
-
Evaluation of Expression in C
C Programs on Operator Precedence and Evaluation
-
Program on Operator Precedence
Conditional Statements
-
Simple if
-
if..else
-
Nested if
-
if .. else Ladder
-
goto Statement
-
Switch Statement
C Programs on Conditional Statements
-
Program on Switch Statement
Loops : while and do..while
-
Introduction to while Loop
-
Infinite while Loop
-
do..while Loop
C Programs on while and do..while Loop
-
Program to Display 1 to N Numbers using while Loop
Loops : for Loop
-
Introduction to for loop
-
for Loop Code Logic Explanation
C Programs on for Loop
-
Program to Display Values from 1 to 10
-
Program to Print the First "N" Numbers
-
Program to Find Factorial of a Given Number
-
Program to Print Sum of Digits of a Positive Number
Loops : Nested Loop
-
Introduction to Nested while Loop
-
Nested while Loop - Code Logic Explanation
-
Introduction to Nested do..while Loop
-
Nested do while Loop - Code Logic Explanation
-
Introduction to Nested for Loop
C Programs on Nested Loops
-
Program on Nested while Loop
-
Program on Nested do..while Loop
-
Write a Nested for Loop Program To print Values from (0,0) to (2,2)
-
Program on Nested for Loop
Loops : Break, Continue and Return Statements
-
Break Statement
-
Continue Statement
-
Return Statement
C Programs on Break, Continue and Return Statements
-
Program on Break, Continue and Return Statements
Arrays
-
Introduction to Arrays
-
Memory Storage of Arrays
-
Declaration of Arrays
-
One Dimensional Array
-
Two Dimensional Arrays
-
Multi Dimensional Arrays
C Programs on Arrays
-
Program to Display Elements in an Array
-
Program to Find Highest and Lowest Elements in Array
Strings and Arrays
-
Declaring an Array as String
-
Initializing an Array with Strings
C Programs on Strings and Arrays
-
Program on Initializing an Array with Strings
-
Program to Read a String
-
Program to Find Length of a String
-
Program to Copying a String
Strings and Arrays : Array of Strings and Multiple Strings
-
Important Statements while using String
-
Alternative use of Input Function
-
Array of Strings
-
Multiple Strings
C Programs on Array of Strings and Multiple Strings
-
Program on Reading Multiple Strings
-
Program to Display Strings and Addresses
Format Specifiers
-
Introduction to String Format Specifiers
-
List of Format Conversions
C Programs on Format Specifiers
-
Using Format Specifiers
-
Reading a String using scanf()
-
Reading a String using getchar()
-
Read line of Text using gets() and puts()
-
Read Line of Text using fgets()
-
Reading a String using gets() and sscanf()
-
Using sscanf(), sprint() and snprintf()
Pointers
-
Introduction to Memories
-
Introduction to Pointers
-
Benefits of using Pointers
-
Concept of Pointers
-
Declaring and Initializing Pointers
-
Points to Remember while using Pointers
-
Pointer to Pointer
-
Void Pointers
C Programs on Pointers
-
Introduction to Operations on Pointers
-
Program on Pointers when they are Pointing to Different Data
-
Program on Increment / Decrement of a Pointer when Pointer Points to an Array
-
Addition/Subtraction of a Constant Number to Pointer
-
Subtraction of One Pointer to Another Pointer
-
Comparison of Two Pointers
Pointer and Strings
-
Introduction to Pointer and Strings
-
Declaration of a Pointer to Handle a String
C Programs on Pointer and Strings
-
Reading a String using Pointer
-
Reading a String with Spaces using Pointer
-
Reading a String using Pointer and Array
-
Copy a String using Pointer
-
Reading a String using Dynamic Memory
Functions
-
Introduction to Functions
-
Function Prototype
-
Defining a Function
-
Calling a Function
C Programs on Functions
-
Introduction to Classification of Functions
-
Function without Arguments and without Return Value
-
Function without Arguments and with Return Value
-
Function with Arguments and with Return Value
-
Function with Arguments and without Return Value
-
Functions Execution During Runtime
Functions : Parameter Passing Techniques
-
Introduction to Parameter Passing Techniques
-
Call by Value
-
Call by Reference
C Programs on Parameter Passing Techniques
-
Program on Call by Value and Call by Reference in Same Function
Functions : Recursive Functions
-
Introduction to Recursive Functions
-
Infinite Recursive Function
-
Direct Recursion
-
Indirect Recursion
C Programs on Recursive Functions
-
Recursion using Static Variable - Code Logic Explanation
-
Recursion using Static Variable - Code Implementation
Storage Class Specifiers
-
Introduction to Storage Class Specifiers
-
Automatic Specifier
-
Register Specifier
-
Static Specifier
-
External Specifier
Scope of Variables
-
Introduction to Scope of Variables
-
Local and Global Variables
Important Statements
-
sizeof Operator
-
Semicolon
-
Comma
-
Blocks
-
ASCII Codes
-
const Keyword
-
Type Casting
Structures
-
Introduction to Structures
-
Defining a Structure
-
Accessing Structure Members
-
Initialization and Declaring Structure Variables
-
Nested Structures
-
Self Referential Structure
Union
-
Introduction to Union
-
Declaring Union
-
Accessing an Union Member
-
Memory Allocation in Union
-
Difference Between Structures and Unions
-
Introduction to Bit Fields
C Programs on Union
-
Program on Bit Fields
Enumerations (enum)
-
Introduction to Enumeration
-
Introduction to Typedef
C Programs on Enumerations
-
Program on Enumerated Data Types
-
Program to Find the Size of Different Variables for Structures
C Programs on Combination of Pointers, Structures, Arrays and Functions
-
Introduction to Combination of Pointers, Structures, Arrays and Functions
-
Arrays and Pointers
-
Pointer to Array
-
Array of Pointers
-
Function with Arrays
-
Function with Strings
-
Passing and Returning Pointer to Functions
-
Pointer to Functions
-
Variable Number of Arguments of a Function
-
Pointer with Structures
-
Structures with Arrays
-
Structures with Functions
Dynamic Memory Allocation
-
Introduction to Memory Allocation
-
Malloc
-
Calloc
-
Realloc
-
Free
Common Mistakes
-
Formatted Input and Output
-
Using Undeclared and UnInitialized Variables
-
Missing Break in Switch Case Statements
-
Subtracting Pointers that do not Refer Same Array
-
Checking Strings Equality Errors
-
Infinite Loops
-
Not Understanding use of Strings and Character Arrays
Problems with Pointers
-
Wild Pointers
-
Introduction to NULL Pointers
-
Introduction to Dangling Pointers
-
Aliasing Pointers
-
Suspicious Pointers
C Programs on Problems with Pointers
-
Program on Null Pointers
-
Program on Dangling Pointers
-
Program on Handling Dangling Pointer with Dynamic Memory
Preprocessor Directives
-
Introduction to Preprocessor Directives
-
File Inclusion
-
Program on File Inclusion
-
Macros Expansion
-
Object-like Macros
-
Function-like Macros
Preprocessor Directives : Conditional Compilation
-
#if – if
-
#ifdef – if defined
-
#endif – end if
-
#else – else
-
#ifndef – if not defined
C Programs on Preprocessor Directives
-
Program - 1 on Preprocessor Directives
-
Program - 2 on Preprocessor Directives
Files
-
Introduction to Files
-
Opening a File
-
Closing a File
-
Types of Files
-
Sequential Files
-
Error Handling in Files
-
Database vs File System
-
Command Line Arguments
C Programs on Files
-
Program on Opening and Closing a File
-
Program on Error Handling in Files
-
Writing to a Text File
-
Reading from a Text File
-
Reading and Writing from a Text File
-
Write the Strings into a File
-
Read the Strings from a File
C Programs on Binary Operations of Files
-
Introduction to Binary Operations on Files
-
Reading and Writing Data to a Binary File
-
Program on Writing and Reading Data to a Binary File
-
Program to Write a Student Data into a File
-
Program to Read a Student Data from a File
-
Program on Writing and Reading to a Binary file using fwrite() and fread()
Files : Random Access Files
-
Introduction to Random Access Files
-
fseek()
-
ftell()
-
rewind()
Graphics
-
Introduction to Graphics
-
Introduction to Graphic Drivers and Modes
C Programs on Graphics
-
Program to Convert Text Mode to Graphic Mode
-
Program to Print Text using Graphics
Graphics : Basic Color Functions
-
textcolor() Function
-
textbackground() Function
-
setcolor(),setbkcolor() and settextstyle() Functions
C Programs on Basic Color Functions using Graphics
-
Program on setcolor(),setbkcolor() and settextstyle() Functions
Graphics : Library Functions
-
Introduction to Graphics Library Functions
-
line()Function
-
circle()Function
-
rectangle()Function
-
bar( )Function
-
ellipse()Function
-
Graphic Functions
C Programs on Library Functions using Graphics
-
Program on All Library Functions
Online Compiler
- This section has no content published in it.